After receiving the Chirs Sells/Ian Griffiths WPF book recently (and having read most of it) I’ve started to make forays into writing WPF code. Its always a bit of a “reality check” when you leave the safe and easy environs of book/SDK demos and start to try and think things up and then build them. Fortunately the book has given me (I think) the conceptual building blocks to create WPF UIs (even if some of the types mentioned in the book seem to have changed). Between the book, the SDKs and the intellisense/feedback VS gives I’ve been able to make my way along slowly.
Tonight I created a fairly simple WPF UI purely in XAML that leverages one of the coolest things I’ve come across thus far in WPF - Data Binding. Binding to an XML data source (statically imbedded in this case, but could easily be loaded from file) and binding properties via XPath is very cool. Here I’m creating the rectangles on the canvas based on the “item“ elements in the Xml data, positioning them based on the attributes in the “position“ element and filling in some text based on the contents of the “title“ and “notes“ elements.
<Window x:Class=“MindPad.XmlDataBinding” xmlns=“http://schemas.microsoft.com/winfx/avalon/2005" xmlns:x=“http://schemas.microsoft.com/winfx/xaml/2005" Title=“MindPad” > <StackPanel xmlns=“http://schemas.microsoft.com/winfx/avalon/2005" xmlns:x=“http://schemas.microsoft.com/winfx/xaml/2005"> <StackPanel.Resources> <XmlDataProvider x:Key=“itemData”> <items xmlns=“”> <item> <position top=“100” left=“50”/> <title>WPF (AKA Avalon)</title> <notes>this little app is made with WPF</notes> <item> <position top=“125” left=“-150”/> <title>Xaml</title> <notes>xml syntax for creating UI</notes> </item> <item> <position top=“20” left=“15”/> <title>Layout</title> <notes>layout is a bit like the web</notes> </item> <item> <position top=“150” left=“175”/> <title>Composition</title> <notes>Composition ROCKS!</notes> </item> </item> </items> </XmlDataProvider> </StackPanel.Resources> <ItemsControl ItemsSource=“{Binding Source={StaticResource itemData}, XPath=//item}”><ItemsControl.Style> <Style TargetType=“{x:Type ItemsControl}”> <Setter Property=“Template”> <Setter.Value> <ControlTemplate TargetType=“{x:Type ItemsControl}”> <Border BorderBrush=“Navy” BorderThickness=“2” CornerRadius=“5”> <Canvas IsItemsHost=“True” Height=“200” Width=“200”> </Canvas> </Border> </ControlTemplate> </Setter.Value> </Setter> </Style> </ItemsControl.Style> <ItemsControl.ItemTemplate> <DataTemplate> <Border BorderBrush=“Green” BorderThickness=“2” CornerRadius=“3” Padding=“3”> <Border.Background> <LinearGradientBrush StartPoint=“0,0” EndPoint=“0, 1”> <LinearGradientBrush.GradientStops> <GradientStop Color=“GreenYellow” Offset=“0” /> <GradientStop Color=“YellowGreen” Offset=“1” /> </LinearGradientBrush.GradientStops> </LinearGradientBrush> </Border.Background> <StackPanel> <TextBlock Text=“{Binding XPath=title/text()}” FontSize=“15” > </TextBlock> <TextBlock Text=“{Binding XPath=notes/text()}” FontSize=“10”> </TextBlock> </StackPanel> </Border> </DataTemplate> </ItemsControl.ItemTemplate> <ItemsControl.ItemContainerStyle> <Style TargetType=“{x:Type ContentControl}”> <Setter Property=“Canvas.Top” Value=“{Binding XPath=position/@top}”/> <Setter Property=“Canvas.Left” Value=“{Binding XPath=position/@left}”/> </Style> </ItemsControl.ItemContainerStyle> </ItemsControl> </StackPanel> </Window>
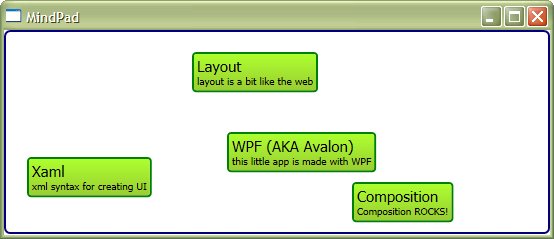